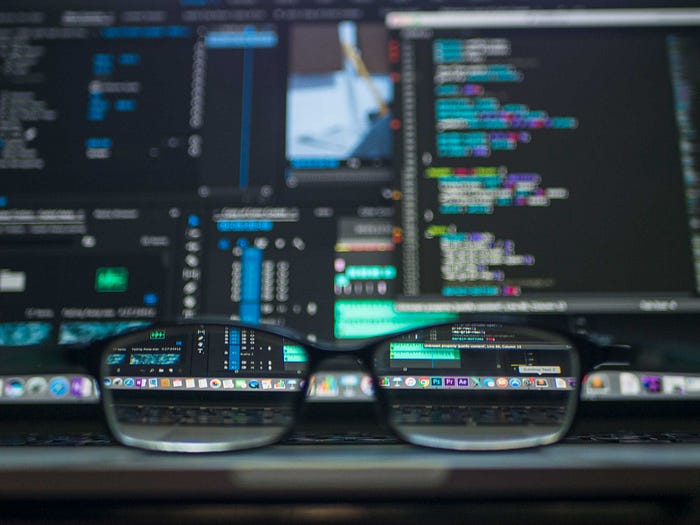
Essential Best Practices for Optimal Performance
Introduction:
PostgreSQL, an open-source relational database management system, has gained immense popularity for its robust features and reliability. To harness its full potential and ensure optimal performance, it’s crucial to follow best practices. In this blog post, we’ll explore key PostgreSQL best practices that can enhance efficiency, maintainability, and scalability.
1. Connection Pooling (Using pg-pool
):
- Efficiently manage database connections using connection pooling.
const { Pool } = require('pg');
const pool = new Pool({
user: 'your_user',
host: 'your_host',
database: 'your_database',
password: 'your_password',
port: 5432,
max: 20, // Set the maximum number of clients in the pool
});
pool.query('SELECT * FROM your_table', (err, res) => {
// Handle query results
});
2. Indexing for Performance:
- Identify and create appropriate indexes to improve query performance.
CREATE INDEX idx_user_email ON users(email);
3. Use of Transactions:
- Wrap multiple SQL statements within a transaction for consistency and atomicity.
const client = await pool.connect();
try {
await client.query('BEGIN');
// Execute SQL statements
await client.query('COMMIT');
} catch (error) {
await client.query('ROLLBACK');
throw error;
} finally {
client.release();
}
4. Stored Procedures:
- Utilize stored procedures for encapsulating business logic on the database side.
CREATE OR REPLACE FUNCTION get_user_by_id(user_id INT) RETURNS SETOF users AS $$
SELECT * FROM users WHERE id = user_id;
$$ LANGUAGE SQL;
5. Full-Text Search (Using tsvector
and tsquery
):
- Implement full-text search capabilities for efficient text searching.
CREATE INDEX idx_user_search ON users USING gin(to_tsvector('english', name));
SELECT * FROM users WHERE to_tsvector('english', name) @@ to_tsquery('english', 'search_term');
6. Data Encryption:
- Use encryption functions to store sensitive information securely.
CREATE EXTENSION IF NOT EXISTS pgcrypto;
INSERT INTO users (username, password)
VALUES ('john_doe', crypt('secure_password', gen_salt('bf')));
7. JSONB Data Type:
- Leverage the JSONB data type for storing and querying JSON data efficiently.
CREATE TABLE products (
id SERIAL PRIMARY KEY,
name VARCHAR(255),
details JSONB
);
INSERT INTO products (name, details)
VALUES ('Laptop', '{"brand": "Dell", "price": 1200}');
8. Partitioning Tables:
- Implement table partitioning for large datasets to improve query performance.
CREATE TABLE logs (
id SERIAL PRIMARY KEY,
log_date DATE,
details JSONB
);
CREATE TABLE logs_january PARTITION OF logs
FOR VALUES FROM ('2022-01-01') TO ('2022-01-31');
9. Database Constraints:
- Define appropriate constraints (e.g., UNIQUE, NOT NULL) to maintain data integrity.
ALTER TABLE users
ADD CONSTRAINT unique_email UNIQUE (email);
10. Materialized Views:
- Use materialized views for precomputed and indexed query results.
CREATE MATERIALIZED VIEW mv_user_counts AS
SELECT role, COUNT(*) AS user_count
FROM users
GROUP BY role;
11. Audit Logging:
- Implement audit logging to track changes made to critical data.
CREATE TABLE audit_log (
id SERIAL PRIMARY KEY,
user_id INT,
action_type VARCHAR(50),
timestamp TIMESTAMP DEFAULT NOW()
);
CREATE FUNCTION log_user_action(user_id INT, action_type VARCHAR(50)) RETURNS VOID AS $$
INSERT INTO audit_log (user_id, action_type) VALUES (user_id, action_type);
$$ LANGUAGE SQL;
12. Database Views:
- Create views to simplify complex queries or present aggregated data.
CREATE VIEW view_user_roles AS
SELECT user_id, role
FROM user_roles;
13. Logical Replication:
- Use logical replication for replicating specific tables or databases.
-- Enable logical replication on the primary server
ALTER SYSTEM SET wal_level = 'logical';
-- Create a replication slot on the primary server
SELECT * FROM pg_create_logical_replication_slot('my_slot', 'pgoutput');
14. Query Optimization:
- Analyze and optimize query performance using
EXPLAIN
andANALYZE
.
EXPLAIN ANALYZE SELECT * FROM users WHERE age > 30;
15. Connection SSL/TLS:
- Enable SSL/TLS connections for secure communication.
const { Pool } = require('pg');
const pool = new Pool({
connectionString: 'your_connection_string',
ssl: {
rejectUnauthorized: false,
},
});
16. Data Masking:
- Implement data masking for sensitive information in query results.
CREATE EXTENSION IF NOT EXISTS pg_masking;
CREATE MASKING POLICY email_masking
ON users FOR SELECT
USING (email || '@example.com' AS email);
17. Foreign Key Constraints:
- Enforce referential integrity using foreign key constraints.
ALTER TABLE orders
ADD CONSTRAINT fk_user_id
FOREIGN KEY (user_id) REFERENCES users(id)
ON DELETE CASCADE;
18. Database Maintenance:
- Regularly perform maintenance tasks like vacuuming to optimize database performance.
VACUUM ANALYZE;
19. Data Archiving:
- Implement data archiving strategies for historical data preservation.
-- Move old records to archive table
INSERT INTO users_archive
SELECT * FROM users WHERE created_at < '2022-01-01';
-- Delete old records from the main table
DELETE FROM users WHERE created_at < '2022-01-01';
20. Database Compression:
- Utilize table compression to reduce storage space.
ALTER TABLE your_table SET (compression = 'pglz');
21. Parallel Query Execution:
- Configure PostgreSQL to allow parallel query execution.
-- Enable parallel query execution
ALTER TABLE your_table SET (parallel_workers = 4);
22. Database Roles and Permissions:
- Create specific roles with limited permissions for better security.
CREATE ROLE app_user LOGIN PASSWORD 'your_password';
GRANT SELECT, INSERT, UPDATE, DELETE ON TABLE your_table TO app_user;
23. Database Monitoring (Using pg_stat_statements
):
- Use
pg_stat_statements
for monitoring and analyzing SQL query performance.
-- Enable the module in postgresql.conf
shared_preload_libraries = 'pg_stat_statements'
-- Query statistics
SELECT * FROM pg_stat_statements;
24. Database Backups:
- Regularly perform database backups and test the restoration process.
pg_dump -U your_user -h your_host -d your_database > backup.sql
25. Data Type Optimization:
- Choose appropriate data types to optimize storage and performance.
-- Use integer instead of bigint if the range is limited
ALTER TABLE your_table ALTER COLUMN column_name TYPE INTEGER;
26. Table Inheritance:
- Explore table inheritance for managing common attributes among tables.
CREATE TABLE vehicles (
id SERIAL PRIMARY KEY,
type VARCHAR(50),
color VARCHAR(50)
);
CREATE TABLE cars () INHERITS (vehicles);
CREATE TABLE trucks () INHERITS (vehicles);
27. Database Extensions:
- Install and use relevant PostgreSQL extensions for additional functionality.
CREATE EXTENSION IF NOT EXISTS "uuid-ossp";
28. Data Replication (Using pg_logical
):
- Implement logical replication for replicating specific data changes.
-- Install the extension
CREATE EXTENSION IF NOT EXISTS pg_logical;
-- Create a replication slot
SELECT pg_create_logical_replication_slot('your_slot', 'pgoutput');
29. Database Version Upgrades:
- Plan and execute database version upgrades carefully.
pg_dumpall > backup.sql
pg_ctl stop
# Upgrade PostgreSQL version
pg_upgrade
30. Database Security Audits:
- Regularly perform security audits and follow best practices for securing PostgreSQL.
-- Review user privileges
SELECT * FROM information_schema.role_table_grants;
Conclusion:
By adhering to these PostgreSQL best practices, you can ensure a high-performing, secure, and scalable database environment for your applications. Regularly review and update your strategies as your application evolves, and leverage the robust features PostgreSQL offers to optimize your database’s performance. Implementing these best practices will not only enhance the efficiency of your PostgreSQL database but also contribute to the overall success of your application.
Relevant Blogs:
https://medium.com/@parmarshyamsinh/the-node-js-best-practices-list-for-2024-18810634c7cc
- Tags:
- #Nodejs
- #PostgreSQL